Angular Dashboard with Front-End API
Table of Contents
In Teragrep, the front-end API limits the binding to a paragraph scope. This restriction avoids Angular object leaks and scope pollution.
Teragrep is currently using v1.5.7 of AngularJS library which support officially ended in January 2022. Read more here. |
Functions
Binding Variables
Use z.angularBind()
and ng-model
to bind a value to both an angular object and a target paragraph.
%angular
<form>
<label class="form-label">What's your name?</label>
<div class="input-group">
<input class="form-control" ng-model="name" type="text" />
<button class="btn btn-primary" ng-click="z.angularBind('name',name,'paragraph_1658728178308_489139183')" type="send">
Send
</button>
</div>
</form>
%angular
Hello {{name}}, nice to meet you!
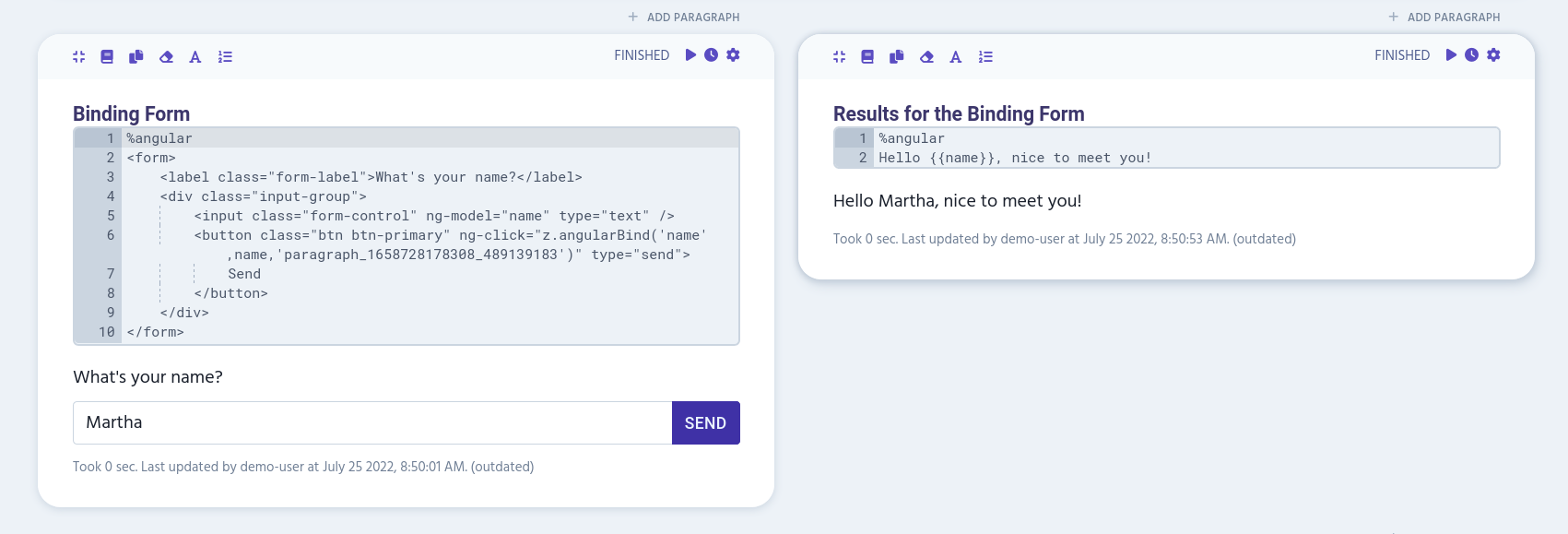
The syntax for z.angularBind()
looks like this:
// all parameters are mandatory
z.angularBind(angularObjectName, angularObjectValue, paragraphID);
Unbinding Variables
Use z.angularUnbind()
to unbind the value from the angular object and the target paragraph.
%angular
<form>
<button type="submit" class="btn btn-primary" ng-click="z.angularUnbind('name','paragraph_1658728178308_489139183')">
Unbind
</button>
</form>
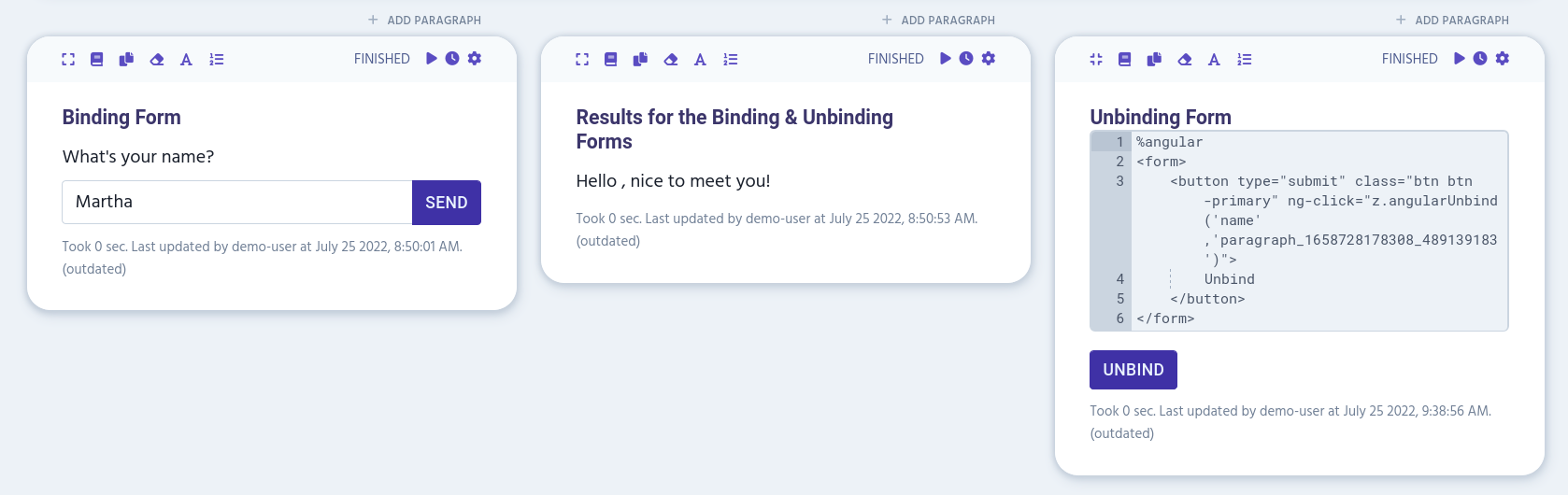
The syntax for z.angularUnbind
looks like this:
// all parameters are mandatory
z.angularUnbind(angularObjectName, angularObjectValue, paragraphID);
Running a Paragraph
Use z.runParagraph()
and ng-model
to create a form, which will run a separate paragraph when its ID is put into the text field.
%angular
<form>
<label class="form-label">Paragraph ID</label>
<div class="input-group">
<input ng-model="id" type="text" class="form-control" placeholder="paragraph_0000000000000_000000000" />
<button ng-click="z.runParagraph(id)" type="submit" class="btn btn-primary">
Run the paragraph
</button>
</div>
</form>
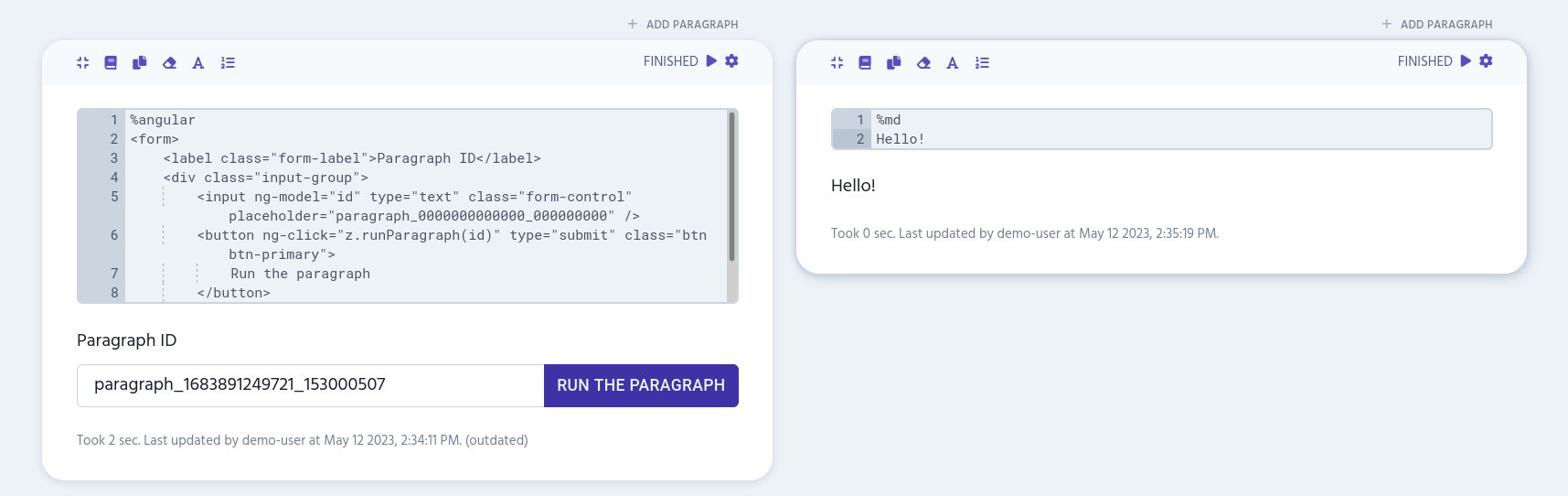
Overriding the Dynamic Form
You can override the dynamic form by using z.angularBind
and the same name in ng-model
as in ${formName}
.
%angular
<form>
<label class="form-label">
Choose your profession
</label>
<div class="input-group">
<input ng-model="professionName" type="text" class="form-control" placeholder="Write the name of profession" />
<button ng-click="z.angularUnbind('professionName', 'paragraphID'); z.runParagraph('paragraphID')" type="submit" class="btn btn-secondary">
Reset
</button>
<button ng-click="z.angularBind('professionName', professionName, 'paragraphID'); z.runParagraph('paragraphID')" type="submit" class="btn btn-primary">
Send
</button>
</div>
</form>
%md
I want to be a ${professionName}!
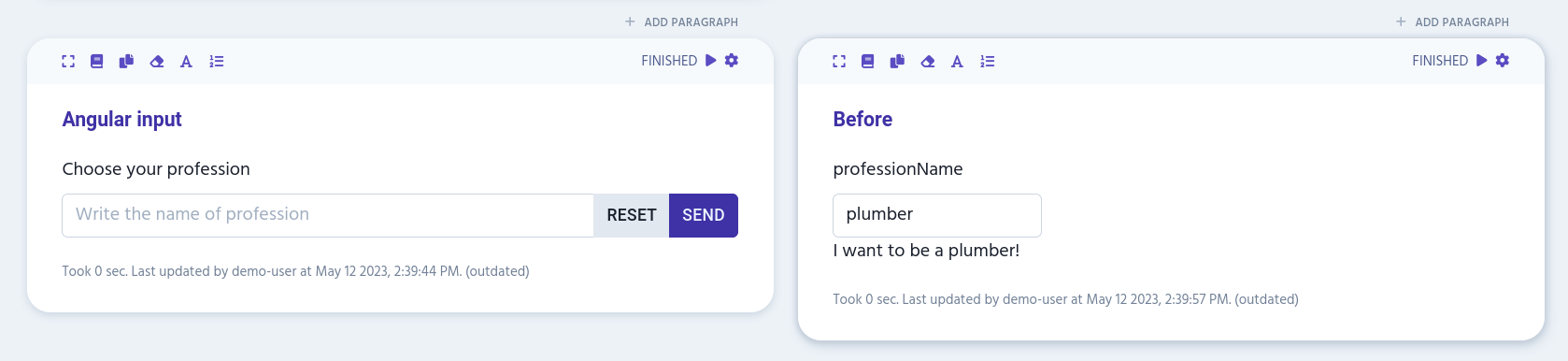
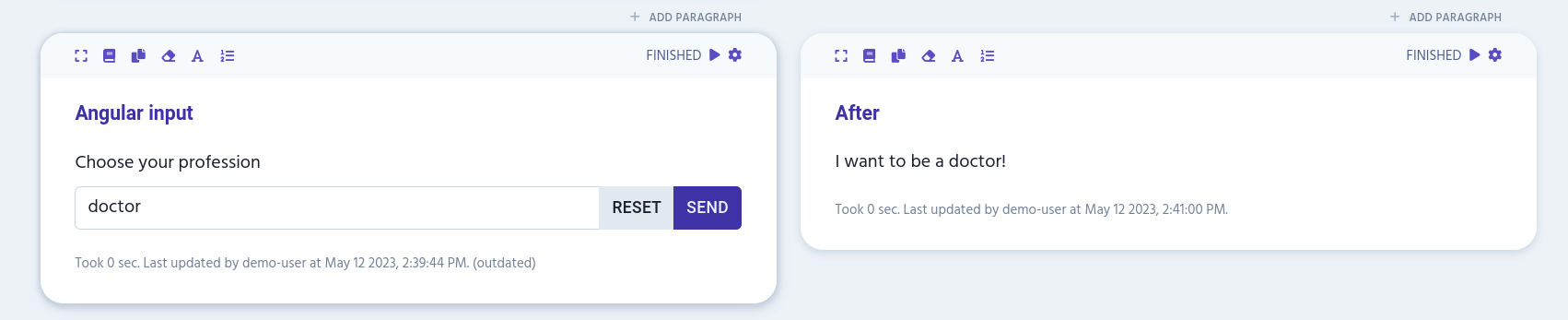